Card
A card is a generic container for grouping related UI elements and content.
Introduction
The Joy UI Card component includes several complementary utility components to handle various use cases:
- Card: a surface-level container for grouping related components.
- Card Overflow: a supplemental wrapper that expands a Card's contents to fill all edges.
- Card Cover: an optional container for displaying background images and gradient layers behind the Card Content.
- Card Content: an optional wrapper that brings content to the front (commonly but not always used with the Card Cover).
- Card Actions: an optional wrapper that groups a set of buttons.
Yosemite National Park is in California's Sierra Nevada mountains.
<Card />
Playground
Basics
import Card from '@mui/joy/Card';
Card is a surface-level container for grouping related components. The demo below shows a typical Card that groups together Typography, Aspect Ratio, and Button components, among others:
Yosemite National Park
April 24 to May 02, 2021
$2,900
Customization
Variants
The Card component supports Joy UI's four global variants: plain
, outlined
(default), soft
, and solid
.
Plain card
Description of the card.
Outlined card (default)
Description of the card.
Soft card
Description of the card.
Solid card
Description of the card.
Sizes
The Card component comes in three sizes: sm
, md
(default), and lg
.
Each size has different padding, gap, and font size values.
Colors
Every palette included in the theme is available via the color
prop.
Play around combining different colors with different variants.
neutral plain card
Inverted colors
When the Card's variant is soft
or solid
, you can use the invertedColors={true}
prop to invert the colors of the children to have enough contrast.
To learn more about this, check out Color Inversion feature.
Gross profit
$ 432.6M
Expand to fill
import CardOverflow from '@mui/joy/CardOverflow';
By default, the Card component adds padding around the outer edges of its contents. To eliminate this white space, add the Card Overflow component inside the Card as a wrapper around the content to be expanded.
Yosemite National Park
California
1 hour ago
Card layers
import CardCover from '@mui/joy/CardCover';
import CardContent from '@mui/joy/CardContent';
The default Card provides a single flat surface for other components to sit on top of. Use the Card Cover and Card Content components to expose multiple layers between a Card and the UI elements on its surface.
Card Cover makes it possible to add images, videos, and color and gradient overlays underneath the Card Content. Hover your mouse on the demo below to see how the layers are stacked:
Card
Card Cover
Card Content
Images and videos
Use an image or a video element inside the Card Cover to display media.
The component uses object-fit: cover
to fill the whole Card by default.
Image
Video
Gradient overlay
To create a gradient overlay—frequently seen when a bright image is used as a background—insert an additional Card Cover component between the image layer and the content layer. You can add any number of Card Covers to create more sophisticated stacked layers this way.
Yosemite National Park
California, USA
Horizontal alignment
Card contents are arranged in a column by default.
For horizontal alignment, add the orientation="horizontal"
prop to the Card.
If present, the Card Overflow component will adapt accordingly.
Yosemite Park
California, USA
Actions
Cards often include actions that users can take, like proceeding to a new page or section of the app. There may be individual (discrete) actions within a given card, or the entire card itself may trigger an action when clicked or tapped.
The following sections explain how to approach each of these scenarios.
Bottom actions
Cards often include buttons at the bottom section. Use CardActions
component as a wrapper of those buttons to create proper spacing around them.
The demo below also use buttonFlex
prop to set the ideal width of the buttons to 120px
while allowing them to shrink if necessary. To learn more about CSS flex, visit MDN's guide.
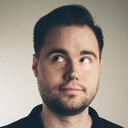
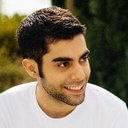
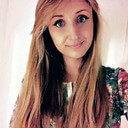
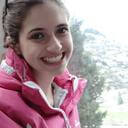
NYC Coders
We are a community of developers prepping for coding interviews, participate, chat with others and get better at interviewing.
Discrete actions
By default, action elements like links and buttons sit above the surface-level interactive area of the Card. In some cases, you might have to adjust the z-index to bring these elements to the front—for instance, the Favorite Icon Button in the demo below needs a higher z-index in order to sit on top of its Aspect Ratio sibling:
Whole Card actions
To make the entire Card clickable, add a Link component to the title (or some other important text content) inside the Card.
Then add the overlay
prop to the Link to spread it across the Card as a whole.
CSS variables playground
Play around with the CSS variables available to the Card component to see how the design changes.
You can use these to customize the component with both the sx
prop and the theme.
Card title
An interesting description.
<Card />
CSS variables
AFSL Web App
Sub project
Next review in 17 days
13
9
32
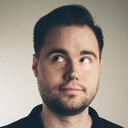
Josephine Blanton
Hello, this is my bio and I am a PRO member of MUI. I am a developer and I love to code.
Add new card
🎊 Congrats Julia 🎊
89
FAQs answered, see if yours is one of them.

Need Some Help?
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor.
$58 /month
Individual License
This license allows you to use the Symbol System Design with unlimited amount of personal and commercial projects.
Professional
- Virtual Credit Cards
- Financial Analytics
- Checking Account
- API Integration
3.990€ / month
Unlimited
- Virtual Credit Cards
- Financial Analytics
- Checking Account
- API Integration
- Cancel Anytime
5.990€ / month
Product card
This example demonstrates the automatic adjustment when a button is placed as a only child of a CardOverflow component. The button will be stretched to fill the entire area of the CardOverflow and the bottom corner radius is also adjusted.
User card
Combining CSS min-width with clamp, a horizontal card can be stacked when it reaches a certain width or below.
The example below shows a user card that stacks when the card's width is equal or below 500px. The drag handle is at the bottom right corner of the card.
Alex Morrison
Senior Journalist
34
980
8.9
Resizable container
This demo uses a technique similar to Heydon Pickering's Flexbox Holy Albatross to create a stretchable Card that switches between vertical and horizontal alignment when its width passes a specified threshold—without using media queries to define breakpoints. Try resizing it by clicking and dragging the bottom-right corner to see how it behaves.
Anatomy
The Card component and all of its supplementary components are composed of a single root <div>
:
<div class="MuiCard-root">
<div class="MuiCardCover-root">
<!-- optional Card Cover layer -->
</div>
<div class="MuiCardContent-root">
<!-- optional Card Content layer -->
</div>
<div class="MuiCardOverflow-root">
<!-- optional Card Overflow utility -->
</div>
<div class="MuiCardActions-root">
<!-- optional Card Actions layer -->
</div>
</div>