Badge
The Badge component generates a small label that is attached to its child element.
Introduction
A badge is a small descriptor for UI elements. It typically sits on or near an element and indicates the status of that element by displaying a number, icon, or other short set of characters.
<Badge />
Playground
Basics
import Badge from '@mui/joy/Badge';
The Badge component wraps around the UI element that it's attached to.
Its default appearance is a dot in the app's primary
color that sits on the top-right corner of the element that it's attached to.
🛒
Content
Use a string or a number as a value for the badgeContent
prop to display content inside the Badge.
🛍
4🔔
❕🪫
Numbers
The following props are useful when badgeContent
is a number.
showZero
By default, the Badge will be automatically hidden when badgeContent={0}
.
You can override this behavior with the showZero
prop:
🛍
0
Maximum value
Use the max
prop to cap the content to a maximum numerical value.
Customization
Variants
The Badge component supports Joy UI's four global variants: solid
(default), soft
, outlined
, and plain
.
💌
1💌
2💌
3💌
4Sizes
The Badge component comes in three sizes: sm
, md
(default), and lg
:
💌
10💌
20💌
30Colors
Every palette included in the theme is available via the color
prop.
💌
P💌
N💌
D💌
S💌
WVisibility
Use the invisible
prop to control the Badge's visibility.
🛍
12Position
By default, the Badge sits on the top-right corner of the element that it's attached to.
Use the anchorOrigin
prop to change the position of the Badge according to its vertical
(top or bottom) and horizontal
(left or right) placement.
Try clicking the arrows in the demo below to change the position of the Badge:
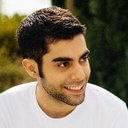
<Badge
anchorOrigin={{
vertical: 'top',
horizontal: 'right',
}}
>
Inset
Use the badgeInset
prop to fine-tune the position of the Badge relative to the element that it's attached to.
This prop accepts a string composed of numbers expressed in units of px
, %
, em
, or rem
. (This syntax corresponds to the inset CSS property.)
This string defines the inset from the Badge's anchorOrigin
—for instance, the demo below pushes the Badge 14% closer to the center of its child element (relative to the top-right corner) along both the vertical and horizontal axes:
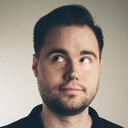
If you pass two unit-numbers to the badgeInset
prop—for example"50px 10px"
—the first number applies to the vertical axis, and the second applies horizontally.
If you pass four unit-numbers to the prop—such as "0 -10px 0 5px"
, they are applied clockwise starting from the top.
Accessibility
Screen readers may not provide users with enough information about a badge's contents.
To make your Badge accessible, you must provide a full description with aria-label
, as shown in the demo below:
Anatomy
The Badge component is composed of a root <span>
that houses the element that the Badge is attached to, followed by a <span>
to represent the Badge itself:
<span class="MuiBadge-root">
<!-- the element the Badge is attached to -->
<span class="MuiBadge-badge">
<!-- Badge content -->
</span>
</span>
Unstyled
Use the Base UI Badge for complete ownership of the component's design, with no Material UI or Joy UI styles to override. This unstyled version of the component is the ideal choice for heavy customization with a smaller bundle size.